Demystifying PyTorch's Image Normalization: Decoding the Mean and Standard Deviation
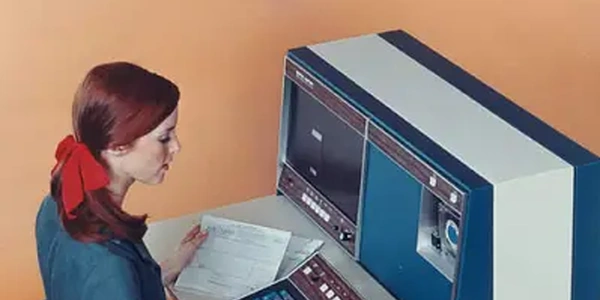
Normalization in Deep Learning
In deep learning, image normalization is a common preprocessing technique that helps improve the training process of neural networks. It involves subtracting the mean pixel intensity (average value) and then dividing by the standard deviation (spread of values) for each color channel (red, green, blue) in an image.
PyTorch's ImageNet Statistics
PyTorch's transforms.Normalize
class uses the following mean and standard deviation values by default:
mean = [0.485, 0.456, 0.406]
std = [0.229, 0.224, 0.225]
These values represent the average pixel intensity and standard deviation of the ImageNet dataset, a massive image collection with millions of labeled images.
Reasons for Using ImageNet Statistics
There are two main reasons PyTorch recommends these values:
-
Pre-trained Models: Many pre-trained models available in PyTorch's
torchvision
library are trained on ImageNet. Using the same normalization statistics ensures the input images are presented in a similar format to what the model was trained on, potentially leading to better performance during fine-tuning on your own dataset. -
Standardization: Normalization helps center the data around zero and scales it to a specific range (often between 0 and 1), which can improve the numerical stability of the training process and sometimes leads to faster convergence.
Important Considerations
- While ImageNet statistics are a good starting point, they might not be optimal for every dataset. If your images have significantly different characteristics, consider calculating your own mean and standard deviation for better results.
- Normalization is just one aspect of image preprocessing. You might also need to perform other operations like resizing and cropping depending on your specific task and model architecture.
In Summary
PyTorch uses the mean and standard deviation values derived from the ImageNet dataset for image normalization because:
- They are a good default for pre-trained models trained on ImageNet.
- Normalization improves the training process by centering and scaling the data.
Remember to evaluate the suitability of these values for your own dataset and adjust accordingly.
Using ImageNet Statistics:
This code snippet demonstrates how to apply image normalization with the default ImageNet statistics:
import torch
from torchvision import transforms
# Define transformations
transform = transforms.Compose([
transforms.ToTensor(), # Convert PIL image to tensor
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225]) # Normalize using ImageNet stats
])
# Load your image (replace with your image loading logic)
img = ... # Load image using PIL
# Apply transformations
normalized_img = transform(img)
Calculating and Using Custom Statistics:
This example shows how to calculate the mean and standard deviation of your own dataset and use them for normalization:
import torch
from torchvision import transforms
# Function to calculate mean and standard deviation (replace with your dataset loading logic)
def calculate_dataset_stats(dataset):
# ... (Implement logic to iterate through your dataset and calculate mean and std)
mean = ...
std = ...
return mean, std
# Load your dataset
dataset = ... # Load your dataset using a PyTorch dataset class
# Calculate statistics
mean, std = calculate_dataset_stats(dataset)
# Define transformations
transform = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize(mean=mean, std=std) # Normalize using custom stats
])
# Load and normalize an image
img = ... # Load image using PIL
normalized_img = transform(img)
Remember to replace the placeholder comments (# ...
) with your specific code for loading images and calculating statistics for your dataset.
Min-Max Scaling:
This approach scales pixel values to a specific range (commonly between 0 and 1).
transform = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize((0.0), (1.0)) # Min-Max scaling (0 to 1)
])
Z-score normalization:
This method centers the data around a mean of 0 and scales it by the standard deviation. It's similar to standard normalization but uses the entire dataset's statistics for calculation.
# Assuming you have calculated mean and std for your dataset
transform = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize(mean=mean, std=std)
])
Local Response Normalization (LRN):
LRN normalizes across local image neighborhoods, which can be helpful for tasks like object detection. PyTorch offers nn.LocalResponseNorm
for this purpose.
transform = transforms.Compose([
transforms.ToTensor(),
# Other transformations
transforms.Lambda(lambda x: nn.functional.local_response_norm(x)) # Apply LRN
])
Choosing the Right Method:
The best method depends on your dataset and model. Here are some general guidelines:
- Normalization (mean-std): Good default for pre-trained models and often improves training stability.
- Min-Max Scaling: Simpler but might not be as effective as normalization for deep learning.
- Z-score normalization: Can be useful if your dataset has a wider range of values compared to ImageNet.
- LRN: More specific to tasks like object detection, can improve feature extraction.
Experimentation is key. Try different methods and evaluate their impact on your model's performance.
python pytorch normalize
Beyond Basics: Exploring Weighted Averages with np.average() in Python
Understanding the Problem:In Python's NumPy library, both np. mean() and np. average() functions are used to calculate the mean (average) of values in an array...
Demystifying the Relationship Between PyTorch and Torch: A Pythonic Leap Forward in Deep Learning
PyTorch and Torch: A Powerful LegacyTorch: Torch is an older deep learning framework originally written in C/C++. It provided a Lua interface...
Troubleshooting "CUDA initialization: CUDA unknown error" in PyTorch
Error Breakdown:CUDA initialization: This part indicates that PyTorch is attempting to initialize its connection with the NVIDIA CUDA toolkit...
Vectorizing PyTorch Snippets for Efficiency: Conquering Two-Dimensional Indirect Indexing
Scenario:Imagine you have a scenario where you want to compute pairwise interactions between elements in a batch of tensors...