Reference Reliance and Dynamic Growth: Navigating Memory in Python's Dynamic World
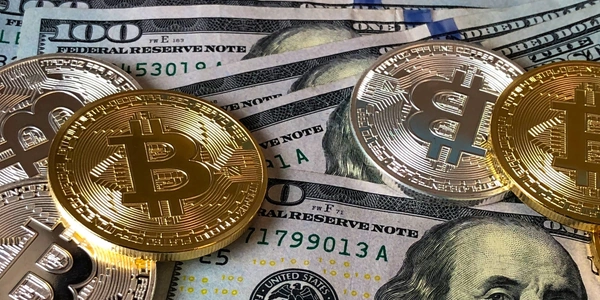
Understanding Memory Usage in Python
In Python, memory usage isn't always straightforward. While you can't precisely measure the exact memory an object consumes, you can make informed approximations using built-in and external tools:
sys.getsizeof() function:
- This built-in function returns the size of the object itself in bytes, excluding any references it holds.
- It's simple but may not capture the complete picture for complex objects.
my_list = [1, 2, 3]
size_of_list = sys.getsizeof(my_list) # Returns size of the list itself, not its elements
size_of_element = sys.getsizeof(my_list[0]) # Returns size of a single element (4 bytes for an integer)
object.__sizeof__() method:
- This method, if implemented by the object's class, can provide a more accurate size estimate.
- Be cautious as custom implementations might not be reliable.
memory_profiler library:
- This external library offers comprehensive memory profiling capabilities.
- It tracks memory allocations and helps identify memory-intensive parts of your code.
from memory_profiler import profile
@profile
def my_function():
# Your memory-intensive code here
my_function() # Run the function and profile its memory usage
Related Issues and Solutions:
Issue: Referenced objects and memory overhead:
sys.getsizeof()
andobject.__sizeof__()
only report the object's direct size, not the memory its referenced objects might occupy.- Consider using
gc.get_referents()
to trace references and estimate memory impact.
Issue: Dynamic memory allocation:
- Data structures like lists and dictionaries grow dynamically, making precise size predictions difficult.
- Monitor memory usage with tools like
memory_profiler
and adjust data structures or algorithms if memory consumption becomes excessive.
Issue: Garbage collection overhead:
- Python's garbage collector manages memory automatically, but it can introduce overhead.
- Understand the garbage collection process and its potential impact on performance.
General Tips:
- Use memory-efficient data structures whenever possible (e.g., use
tuple
instead oflist
for immutable data). - Profile your code regularly to identify memory bottlenecks.
- Consider using object pools or memory caches for frequently accessed data.
By following these guidelines and using the appropriate tools, you can gain valuable insights into memory usage in your Python applications and make informed decisions to optimize performance.
python performance memory-profiling
GET It Right: Mastering Data Retrieval from GET Requests in Django
Understanding GET Requests and Query StringsIn Django, GET requests are used to send data from a web browser to your web application along with the URL...
Python: One-Line Conditional Statements with Conditional Expressions (Ternary Operator)
I'd be glad to explain how to put a simple if-then-else statement on one line in Python:Conditional Expressions (Ternary Operator):...
From Beginner to Ninja: Taming Python Lists with del , remove , and pop
del:A keyword, not a function.Removes elements by index.Doesn't return the removed element.Example:remove(value):A list method...
Plot Perfect: A Recipe for Adding Stellar Labels to Your Pandas Visualizations
Understanding the Problem:In Python, when you create visualizations using pandas, providing clear x and y labels is crucial for informing viewers about the data being represented...