Multi-Level Magic: Unveiling the Secrets of Sorting by Two or More Columns in pandas
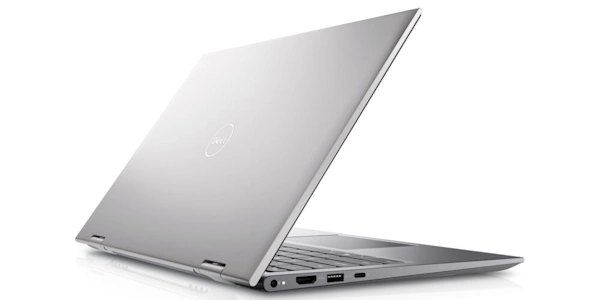
Understanding DataFrames and Sorting:
- DataFrames: Imagine a spreadsheet where data is organized in rows and columns. Each row represents an observation (like a person's information), and each column represents a variable (like name, age, or city). Pandas DataFrames store this tabular data.
- Sorting: Arranging data in a specific order based on one or more columns. This helps visualize patterns, compare values, and find specific information more easily.
Sorting by Multiple Columns:
- Using sort_values():
- This method is key for sorting DataFrames.
- Specify the columns to sort by using the
by
argument and a list of column names. - Control sorting order (ascending or descending) using the
ascending
argument (a list of booleans).
Example:
import pandas as pd
data = {'Name': ['foo', 'bar', 'Charlie', 'David'],
'Age': [25, 30, 28, 35],
'City': ['New York', 'Los Angeles', 'Chicago', 'Houston']}
df = pd.DataFrame(data)
# Sort by Age in ascending order, then Name in ascending order
df_sorted = df.sort_values(by=['Age', 'Name'], ascending=[True, True])
print(df_sorted)
Output:
Name Age City
0 foo 25 New York
2 Charlie 28 Chicago
1 bar 30 Los Angeles
3 David 35 Houston
Key Points:
- Sorting prioritizes columns in the order you specify in the
by
argument. - The
ascending
argument defaults toTrue
(ascending order) for all columns if not specified. - Use a single boolean value in
ascending
to apply the same order to all columns.
Additional Tips:
- To modify the original DataFrame in-place, set
inplace=True
withinsort_values()
. - Use
ignore_index=True
to reset the index after sorting.
Troubleshooting:
- Check for typos in column names.
- Ensure data types are compatible for sorting (e.g., convert strings to numbers if needed).
python pandas python-2.7
Enforcing Maximum Values for Numbers in Django: Validators vs. Constraints
Methods:There are two primary approaches to achieve this:Using Validators: Django provides built-in validators that you can leverage on your model fields...
Conquering Commonality: Efficiently Unearthing the Most Frequent Value in NumPy
Understanding the Problem:In Python, NumPy (Numerical Python) is a powerful library for numerical computing. One common task is to analyze data stored in NumPy arrays...
Peek Inside Your Excel File: Mastering Sheet Name Retrieval with Pandas
Understanding the Problem:Imagine you have an Excel file with multiple sheets, and you want to know the names of each sheet before diving into the data...
Pythonic Techniques for Traversing Layers in PyTorch: Essential Skills for Deep Learning
Iterating Through Layers in PyTorch Neural NetworksIn PyTorch, neural networks are built by composing individual layers...