Step-by-Step Guide to Updating SQLAlchemy Rows with Clarity
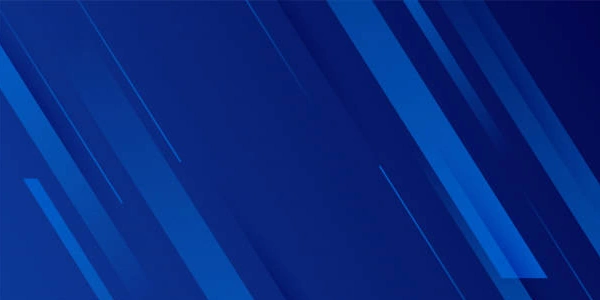
Understanding the Problem:
- Goal: Modify existing data within a database table.
- Tools: Python, SQLAlchemy (an ORM), and potentially Flask-SQLAlchemy (for web applications).
Methods to Update Rows:
-
Updating Objects Directly:
- Retrieve the object you want to modify:
user = User.query.filter_by(username='johndoe').first()
- Change its attributes:
user.email = '[email protected]'
- Commit the changes to the database:
db.session.commit()
- Retrieve the object you want to modify:
-
Using the update() Method:
- Construct an update query:
query = User.query.filter_by(username='johndoe') query.update({'email': '[email protected]'}) db.session.commit()
- Construct an update query:
-
Querying and Updating in a Single Step:
- Combine filtering and updating:
db.session.query(User).filter_by(username='johndoe').update({'email': '[email protected]'}) db.session.commit()
- Combine filtering and updating:
-
Using the Core Expression Language:
- For more complex updates:
from sqlalchemy import update stmt = update(User).where(User.username == 'johndoe').values(email='[email protected]') db.session.execute(stmt) db.session.commit()
- For more complex updates:
Key Points:
- Import necessary modules:
from sqlalchemy import create_engine, Column, Integer, String
- Establish database connection:
engine = create_engine('your_database_url')
- Define models (if using ORM):
class User(db.Model): ...
- Retrieve objects for modification (methods 1, 3, and 4):
user = User.query.filter_by(...).first()
- Construct update queries (update() method):
query = User.query.filter_by(...).update({...})
- Commit changes to the database:
db.session.commit()
Remember:
- Clarity: Provide clear explanations and code examples.
- Beginner-friendly: Use simple language and avoid jargon.
- Context: Explain the purpose of each step.
- Best practices: Highlight recommended approaches.
- Common pitfalls: Address potential errors or mistakes.
python sqlalchemy flask-sqlalchemy
Level Up Your Data Wrangling: Splitting Pandas Dates into Month and Year
Problem:Imagine you have a table of data in Python, managed using a pandas DataFrame. One of the columns contains dates and times...
Understanding Bi-Directional Relationships in SQLAlchemy with backref and back_populates
Relationships in SQLAlchemySQLAlchemy, a popular Python object-relational mapper (ORM), allows you to model database relationships between tables using classes...
Streamlining Data Analysis: Python's Pandas Library and the Art of Merging
Pandas Merging 101In Python's Pandas library, merging is a fundamental technique for combining data from two or more DataFrames (tabular data structures) into a single DataFrame...
Taming TensorBoard Troubles: Effective Solutions for PyTorch Integration
Understanding the Components:Python: A general-purpose programming language widely used in machine learning due to its readability...