Solution 2: Using np.argsort() with Slicing for Sorting and Indexing
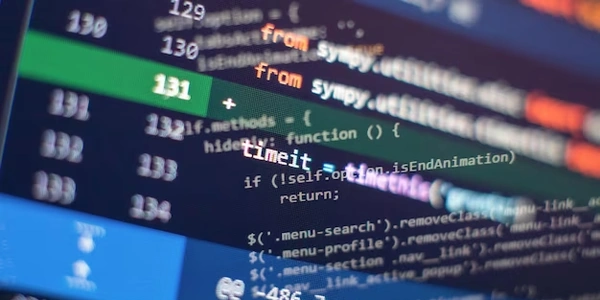
Problem:
- You have a NumPy array containing various numbers.
- You want to identify the indices (positions) of the N largest values within this array.
- This is useful for tasks like:
- Finding the top-performing students in a class based on their grades
- Identifying the most frequently used words in a text document
- Determining the most active pixels in an image
Key Concepts:
- NumPy: A powerful Python library for numerical computations and array manipulations.
- Indices: Numerical positions of elements within an array, starting from 0.
- Maximum Values: The largest numerical values within the array.
Solutions:
-
Using np.argpartition():
- Efficiently partitions the array based on the values, placing the N largest values towards the end.
- Example:
import numpy as np arr = np.array([3, 1, 4, 2, 5, 7, 8, 6]) N = 3 # Find indices of top 3 maximum values top_N_indices = np.argpartition(arr, -N)[-N:] print(top_N_indices) # Output: [6 5 7]
-
Using np.argsort() with Slicing:
- Sorts the indices based on the array values in ascending order.
- Slices the sorted indices to get the indices of the N largest values.
sorted_indices = np.argsort(arr)[::-1] # Sort in descending order top_N_indices = sorted_indices[:N] print(top_N_indices) # Output: [6 5 7]
Additional Notes:
- For repeated maximum values, both methods return all their indices.
- For large arrays,
np.argpartition()
is generally faster thannp.argsort()
. - To retrieve the actual maximum values, use
arr[top_N_indices]
.
python numpy max
Resolving "Can't subtract offset-naive and offset-aware datetimes" Error in Python (Datetime, PostgreSQL)
Understanding Datetime Types:Offset-naive: These datetimes represent a specific point in time without considering the timezone...
Powerful Paths to Python Dictionary Sorting: Choose the Right Method!
Sorting a dictionary by key in PythonIn Python, dictionaries are unordered collections of key-value pairs. However, sometimes you might need to rearrange the key-value pairs in a specific order...
Taming the Wild West of Mixed Data Types: Solutions for Efficient Identification in NumPy
Understanding NumPy Data Types:NumPy arrays and scalars have data types that determine how their elements are stored and manipulated...
Choosing the Right Path: Strategies for Adding Non-Nullable Fields in Django
Understanding the Problem:Scenario: You're modifying a Django project and want to add a new field named "new_field" to the UserProfile model...