Peeking Under the Hood: How to Get the Learning Rate in PyTorch
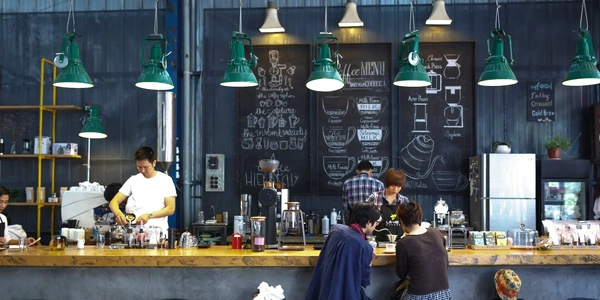
Understanding Learning Rate in Deep Learning
In deep learning, the learning rate is a crucial hyperparameter that controls how much the model's weights are adjusted based on the errors (gradients) calculated during training. A proper learning rate helps the model converge effectively to the optimal weights that minimize the loss function.
Obtaining the Learning Rate in PyTorch
There are two primary scenarios to consider when retrieving the learning rate in PyTorch:
-
Using a Constant Learning Rate:
- If you're employing a constant learning rate throughout training (not recommended for most cases), you can directly access the
lr
attribute of the optimizer you've created. Here's an example:
import torch.optim as optim # ... (model definition and data loading) optimizer = optim.SGD(model.parameters(), lr=0.01) # Set learning rate during optimizer creation for epoch in range(num_epochs): # ... (training loop) learning_rate = optimizer.lr # Access the constant learning rate print(f"Epoch: {epoch+1}, Learning Rate: {learning_rate}")
- If you're employing a constant learning rate throughout training (not recommended for most cases), you can directly access the
-
Using a Learning Rate Scheduler:
- For more sophisticated training, you'll likely incorporate a learning rate scheduler that adjusts the learning rate dynamically based on factors like the number of epochs or the loss value. PyTorch offers various learning rate schedulers (
torch.optim.lr_scheduler
).
In this case, to retrieve the current learning rate after the scheduler has updated it:
import torch.optim as optim from torch.optim.lr_scheduler import ReduceLROnPlateau # ... (model definition and data loading) optimizer = optim.SGD(model.parameters(), lr=0.1) scheduler = ReduceLROnPlateau(optimizer, factor=0.5, patience=2) # Example scheduler for epoch in range(num_epochs): # ... (training loop) # Update learning rate after each epoch (or other criteria) scheduler.step() # Access the current learning rate after the scheduler's update learning_rate = scheduler.get_lr()[0] # Assuming a single learning rate group print(f"Epoch: {epoch+1}, Learning Rate: {learning_rate}")
- The
scheduler.get_lr()
method returns a list containing the current learning rates for all parameter groups (if applicable). In most cases, you'll have a single learning rate group, so you can access the first element ([0]
).
- For more sophisticated training, you'll likely incorporate a learning rate scheduler that adjusts the learning rate dynamically based on factors like the number of epochs or the loss value. PyTorch offers various learning rate schedulers (
Key Points:
- For constant learning rates, directly access the
optimizer.lr
attribute. - For learning rate schedulers, use
scheduler.get_lr()
after the scheduler updates the learning rate. - Consider using learning rate schedulers to improve training performance.
By effectively monitoring and adjusting the learning rate, you can optimize your deep learning models in PyTorch.
Constant Learning Rate:
import torch
import torch.nn as nn
import torch.optim as optim
# Define a simple model (replace with your actual model)
class MyModel(nn.Module):
def __init__(self):
super(MyModel, self).__init__()
self.linear = nn.Linear(10, 1)
def forward(self, x):
return self.linear(x)
# Create some sample data
x = torch.randn(10, 10)
y = torch.randn(10, 1)
# Define the model and optimizer with a constant learning rate
model = MyModel()
optimizer = optim.SGD(model.parameters(), lr=0.01) # Set constant learning rate
# Training loop (simplified)
for epoch in range(2):
# ... (training operations)
# Access and print the constant learning rate
learning_rate = optimizer.lr
print(f"Epoch: {epoch+1}, Learning Rate: {learning_rate}")
Learning Rate Scheduler (ReduceLROnPlateau):
import torch
import torch.nn as nn
import torch.optim as optim
from torch.optim.lr_scheduler import ReduceLROnPlateau
# Define a simple model (replace with your actual model)
class MyModel(nn.Module):
def __init__(self):
super(MyModel, self).__init__()
self.linear = nn.Linear(10, 1)
def forward(self, x):
return self.linear(x)
# Create some sample data
x = torch.randn(10, 10)
y = torch.randn(10, 1)
# Define the model and optimizer
model = MyModel()
optimizer = optim.SGD(model.parameters(), lr=0.1)
# Create a learning rate scheduler that reduces LR on plateau
scheduler = ReduceLROnPlateau(optimizer, factor=0.5, patience=2)
# Training loop (simplified)
for epoch in range(5):
# ... (training operations)
# Update learning rate after each epoch (or other criteria)
scheduler.step() # This is where the learning rate might be adjusted
# Access the current learning rate after the scheduler's update
learning_rate = scheduler.get_lr()[0] # Assuming a single learning rate group
print(f"Epoch: {epoch+1}, Learning Rate: {learning_rate}")
These examples demonstrate how to retrieve the learning rate during training in PyTorch, both for constant values and when using a learning rate scheduler. Remember to replace the sample model and data with your actual deep learning setup.
Custom Callback Function:
- Create a custom callback function that gets called at specific points during training (e.g., after each epoch).
- Inside the callback function, access the optimizer's learning rate or the scheduler's current learning rate (depending on your setup).
- This method offers flexibility to log or perform other actions based on the learning rate.
Example:
import torch
import torch.optim as optim
def print_learning_rate(optimizer):
"""Custom callback to print learning rate."""
print(f"Current Learning Rate: {optimizer.lr}")
# ... (model and optimizer definition)
for epoch in range(num_epochs):
# ... (training loop)
print_learning_rate(optimizer) # Call the callback after each epoch
# ...
TensorBoard Logging (if using TensorBoard):
- If you're using TensorBoard for visualization, you can log the learning rate as a scalar during training.
- Access the learning rate as in the previous methods and use
writer.add_scalar
within your training loop.
Example (assuming TensorBoard setup):
from torch.utils.tensorboard import SummaryWriter
# ... (model, optimizer, and data loader definition)
writer = SummaryWriter()
for epoch in range(num_epochs):
# ... (training loop)
learning_rate = optimizer.lr # Or scheduler.get_lr()[0]
writer.add_scalar("Learning Rate", learning_rate, epoch) # Log learning rate
# ...
Remember to choose the method that best suits your training setup and logging needs. Using a learning rate scheduler is generally recommended for optimal training, but constant learning rates or custom callbacks might be suitable in specific scenarios.
python machine-learning deep-learning