Encapsulation in Python: Protecting Your Code's Secrets (the Right Way)
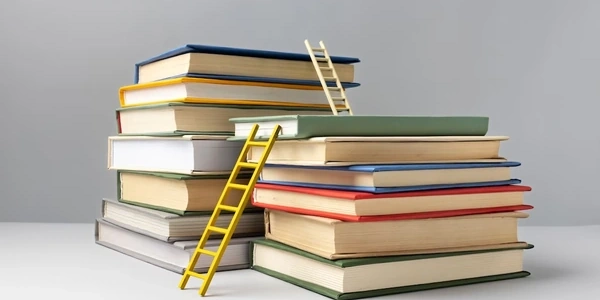
Here's why these methods aren't truly private, and why it's still important to use this convention:
The Name Mangling Trick:
While you can't access a "private" method directly using its original name from outside the class, Python performs a trick called name mangling. It modifies the name behind the scenes to make it appear different.
For example, if you have a method named __secret_method
inside your class, its mangled name becomes something like _ClassName__secret_method
. This mangled name is not directly accessible from outside the class.
However, you can still access the method using the mangled name:
class MyClass:
def __init__(self):
self.__secret_data = "This is secret!"
def __secret_method(self):
print(f"Accessing secret data: {self.__secret_data}")
# This will work, but it's strongly discouraged!
obj = MyClass()
obj._MyClass__secret_method() # Accessing the "private" method
Why Use "Private" Methods Even Though They're Not Truly Private?
Even though you can technically bypass the name mangling, using the double underscore naming convention is still essential for several reasons:
- Encapsulation and Information Hiding: It encourages good programming practices like encapsulation, which involves bundling data and the methods that operate on it within a class. This helps to hide the internal implementation details and promotes better code organization.
- Clarity and Code Readability: Using double underscores for internal methods signals to other developers that these methods are not intended for external use. This improves code readability and avoids confusion about which methods are part of the public interface.
- Future-Proofing: While currently, accessing "private" methods is discouraged, future versions of Python may introduce stricter privacy mechanisms that respect the double underscore convention. By following this practice, you ensure your code adapts seamlessly to potential changes.
Alternatives for True Privacy (if needed):
In situations where absolute privacy is crucial, Python offers alternative approaches, although they're not as straightforward as true private methods:
- Properties: These provide controlled access to attributes and can be used to restrict modification or access.
- Closures: By creating functions within functions and returning only specific parts, you can achieve a level of data privacy.
Remember: Even with these alternatives, relying solely on language mechanisms for tight data protection is not always advisable. It's essential to design your classes and functions with security and proper access control in mind.
python encapsulation information-hiding
Determining an Object's Class in Python: Methods and When to Use Them
Getting the Class Name of an InstanceIn Python, you can determine the class an object belongs to by accessing its special attributes:...
Demystifying Legend Placement: Move It Outside Your Plot with Matplotlib and Seaborn
Here's a breakdown of how to achieve this in Python using Matplotlib and Seaborn, along with sample codes and related solutions:...
Choosing Your Weapon: Selecting the Best Method to Remove Duplicate Columns in pandas
Understanding Duplicate Columns:In a pandas DataFrame, duplicate columns refer to those that have identical values in all rows...
Taming the ValueError: Effective Ways to Check for None or NumPy Arrays
Understanding the Error:In Python, you'll encounter a ValueError when you try to use the not operator on a NumPy array in a conditional statement like if...