Beyond TensorFlow: When and Why to Convert Tensors to NumPy Arrays for Enhanced Functionality
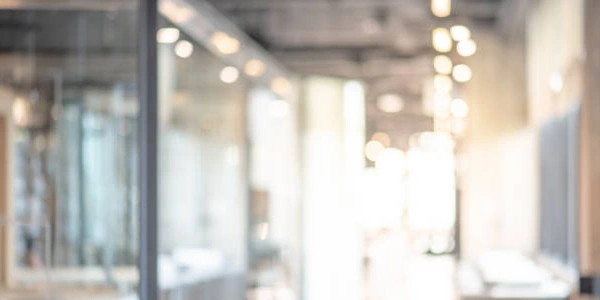
Understanding Tensors and NumPy Arrays:
- Tensors: These are the fundamental data structures in TensorFlow, used for numerical computations and representing multi-dimensional arrays.
- NumPy Arrays: These are powerful arrays in Python, offering efficient operations and a rich set of mathematical functions.
Why Convert Tensors to NumPy Arrays?
- Accessing NumPy's Functionality: NumPy has a vast library of functions for data manipulation, linear algebra, and more. Converting tensors to NumPy arrays gives you access to these tools.
- Visualization and Analysis: Many visualization libraries like Matplotlib and Seaborn work seamlessly with NumPy arrays, making it easier to visualize and analyze data.
- Integration with Other Python Libraries: NumPy arrays are widely used in Python's scientific ecosystem, allowing smooth integration with other libraries.
Conversion Methods:
-
Using the .numpy() Method:
-
Directly call the
numpy()
method on a tensor to get its NumPy array representation. -
Example:
import tensorflow as tf tensor = tf.constant([[1, 2, 3], [4, 5, 6]]) numpy_array = tensor.numpy() print(numpy_array) # Output: [[1 2 3] # [4 5 6]]
-
-
Using tf.make_ndarray():
-
Create a NumPy array from a tensor using the
tf.make_ndarray()
function. -
Example:
numpy_array = tf.make_ndarray(tensor) print(numpy_array) # Same output as above
-
Important Considerations:
- GPU Memory: Tensors can reside on GPU memory for accelerated computations. Converting them to NumPy arrays involves a memory copy to CPU memory, which can be slow for large tensors.
- Eager Execution: In TensorFlow's eager execution mode (default in recent versions),
.numpy()
andtf.make_ndarray()
are generally efficient. - Graph Execution (TensorFlow 1.x): In graph execution mode, explicitly evaluating tensors within a session might be necessary using
tf.Session.run()
.
Related Issues and Solutions:
- Tensors on GPU: If the tensor is on GPU memory and you need to work with it on the CPU, transfer it to CPU memory first using
tf.config.set_visible_devices()
orwith tf.device('/cpu:0'):
. - Errors in Graph Execution: If you encounter errors in graph execution mode, ensure the tensor has been evaluated within a session using
tf.Session.run()
.
Remember: Choose the conversion method based on your TensorFlow version and execution mode for optimal performance.
python numpy tensorflow
Beyond the Basics: Addressing Challenges in Retrieving Module Paths
Methods to Retrieve Module Path:Using the __file__ attribute: This built-in attribute within a module holds the absolute path to the module's file...
Iterating Over Defined Columns in SQLAlchemy Models (Python)
I'd be glad to explain how to iterate over the defined columns of a SQLAlchemy model in Python:Iterating Through SQLAlchemy Model Columns...
Resolving Common Challenges: Nested Data, Type Mismatches, and Django Integration
Understanding the Problem:JSON (JavaScript Object Notation): It's a widely used format for exchanging data between systems...
Data Transformation Made Easy: Converting Lists to Pandas DataFrames with Confidence
Understanding the Problem:You have a list containing two sublists: The first sublist represents the column headers (labels for each data point)...