Flexibility or Static Reference? Choosing the Right Approach for User Models in Django
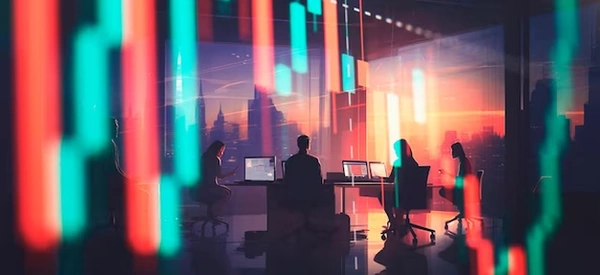
Understanding User Models in Django:
- Django's authentication system revolves around the "User" model, which stores user data like usernames, passwords, and related attributes.
- By default, Django provides a basic
User
model, but you can create a custom user model tailored to your application's needs. - When using a custom user model, the way you reference and interact with it changes, and that's where
get_user_model
andsettings.AUTH_USER_MODEL
come in.
Key Differences and Use Cases:
Feature | get_user_model() | settings.AUTH_USER_MODEL |
---|---|---|
Purpose | Retrieving the active user model at runtime, handling potential custom models. | Specifying the active user model in settings, mainly for static references. |
Use Cases | - Model definitions (foreign keys, relations) | - Migrations |
- Code that needs to be flexible across projects with different user models | - Custom middleware & signals | |
- Reusable apps/libraries interacting with user models | - Views/templates needing static user model reference | |
Syntax | from django.contrib.auth import get_user_model; User = get_user_model() | AUTH_USER_MODEL = 'myapp.MyCustomUser' |
Flexibility | Dynamic, adapts to custom models | Static, requires restart for changes |
Best Practices and Examples:
-
Using get_user_model() in Model Definitions:
from django.contrib.auth import get_user_model class Author(models.Model): user = models.ForeignKey(get_user_model(), on_delete=models.CASCADE) # ... other fields # This ensures flexibility, even if a custom user model is used in the future.
-
Using settings.AUTH_USER_MODEL in Migrations:
from django.db import migrations, models def create_initial_data(apps, schema_editor): MyCustomUser = apps.get_model('myapp', 'MyCustomUser') # Create initial user instances using MyCustomUser migrations.CreateModel( name='MyModel', fields=[ ('user', models.ForeignKey(to=settings.AUTH_USER_MODEL, on_delete=models.CASCADE)), # ... other fields ], ) class Migration(migrations.Migration): dependencies = [ ('auth', '0012_alter_user_first_name_max_length'), ] operations = [ migrations.RunPython(create_initial_data), ]
# After changing AUTH_USER_MODEL, apply migrations: python manage.py makemigrations python manage.py migrate
-
Using Both Dynamically in Views/Templates:
from django.contrib.auth import get_user_model def some_view(request): User = get_user_model() # Retrieve dynamically context = {'users': User.objects.all()} # Query using the retrieved model return render(request, 'some_template.html', context)
{% for user in users %} <h2>{{ user.username }}</h2> {% endfor %}
Common Issues and Solutions:
- Circular dependency issues: Use
from ... import User
sparingly, preferget_user_model()
or explicit imports. - Incorrect field references: When using a custom model, ensure you're accessing fields correctly via the retrieved model class.
- Migration errors: Always migrate after changing
AUTH_USER_MODEL
to apply model changes to the database.
By understanding these distinctions and following best practices, you can write robust Django applications that can seamlessly adapt to custom user models and ensure maintainability in the long run.
python django
Bound Methods in Python: Understanding the "self" and Object Interaction
Bound Methods:These are the most common type of methods. They are attached to a specific object (instance) of the class and can access the object's attributes using the self keyword...
Serializing Django Model Instances: Understanding the Options
Serialization in DjangoSerialization is the process of converting a complex data structure (like a Django model instance) into a format that can be easily transmitted or stored...
Grabbing IDs After Inserts: flush() and Strategies in SQLAlchemy (Python)
SQLAlchemy flush()In SQLAlchemy, a session acts as a buffer between your Python objects and the underlying database.When you create a new object and add it to the session using session...
Data Wrangling Zen: The Art of Calm and Informed Analysis with Pandas Progress
Uncertainty: Waiting without knowing the progress can be anxiety-inducing, especially with large datasets.Debugging: Unresponsive operations make it hard to identify bottlenecks or errors...