Demystifying Data Conversion: Converting Strings to Numbers in Python
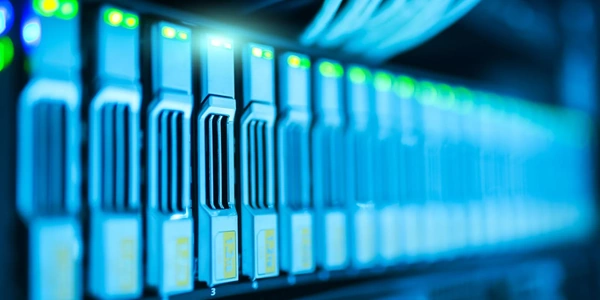
Parsing in Python refers to the process of converting a string representation of a value into a different data type, such as a number. In this case, you're converting a string to a numerical data type, either a floating-point number (float) or an integer (int).
Python provides built-in functions to handle these conversions:
- int(string): This function attempts to convert the
string
argument to an integer. It will only succeed if the entire string consists of digits (0-9) optionally preceded by a plus or minus sign (+
or-
). - float(string): This function attempts to convert the
string
argument to a floating-point number. It will succeed if the string is a valid representation of a decimal number, which can include a decimal point and an optional exponent.
Here's why floating-point numbers are relevant:
Floating-point numbers are a data type in Python that represent numbers with decimal points. They are used when you need to store numbers with a fractional component, such as scientific measurements or financial calculations.
Example:
number_string = "123.45"
# Parse the string to a float
float_number = float(number_string)
print(float_number) # Output: 123.45
another_string = "hello"
# Attempt to parse a non-numeric string to a float (will result in ValueError)
try:
float_result = float(another_string)
except ValueError:
print("Could not convert", another_string, "to a number")
Important considerations:
- If the string you're trying to parse doesn't follow the expected format (e.g., contains characters other than digits, ., +, or -), the
int()
orfloat()
functions will raise aValueError
. - It's good practice to use a
try-except
block to handle potential parsing errors. This way, your code can gracefully handle invalid input and avoid crashing.
I hope this explanation clarifies how to parse strings to floats or ints in Python!
Example 1: Simple parsing
# Parse string to integer if possible
number_string = "100"
integer_value = int(number_string)
print("Integer:", integer_value) # Output: Integer: 100
# Parse string to float if possible
decimal_string = "3.14"
float_value = float(decimal_string)
print("Float:", float_value) # Output: Float: 3.14
Example 2: Handling errors with try-except
# Attempt to parse a string, handle potential ValueError
number_string = "hello"
try:
integer_value = int(number_string)
print("Converted to integer:", integer_value)
except ValueError:
print("Could not convert", number_string, "to an integer")
# Parse a valid float string
decimal_string = "2.71"
float_value = float(decimal_string)
print("Float:", float_value) # Output: Could not convert hello to an integer
# Float: 2.71
Example 3: Parsing with a custom function
def parse_number(string):
"""
Attempts to parse a string to a float, then to an integer if float fails.
Args:
string: The string to parse.
Returns:
The parsed number (float or int) or None if parsing fails.
"""
try:
return float(string)
except ValueError:
try:
return int(string)
except ValueError:
return None
user_input = input("Enter a number: ")
parsed_value = parse_number(user_input)
if parsed_value is not None:
print("Parsed number:", parsed_value)
else:
print("Invalid input. Please enter a number.")
These examples showcase different approaches to parsing strings to numbers in Python. You can choose the method that best suits your specific needs and error handling requirements.
eval() (use with caution):
The eval()
function evaluates a string as Python code. You can use it to convert a string like "10 + 5"
to the number 15. However, this is generally not recommended due to security risks. Malicious input could potentially execute arbitrary code in your program. Only use eval()
in controlled environments where you trust the source of the string.
Regular expressions (advanced):
Regular expressions are powerful tools for pattern matching in strings. You can use them to check if a string adheres to a specific format (e.g., digits with an optional decimal point) before attempting conversion with int()
or float()
. This approach offers more control over what constitutes a valid number string, but it requires a deeper understanding of regular expressions.
ast.literal_eval() (limited use):
The ast.literal_eval()
function from the ast
module is a safer alternative to eval()
for simple literal evaluations. However, it's limited to basic numeric literals, strings, lists, tuples, and dictionaries. It won't work for more complex expressions.
Libraries like decimal or fractions:
For specific use cases involving high-precision decimal calculations, libraries like decimal
or fractions
provide specialized data types for handling decimals with greater accuracy than native float
types. These libraries offer methods for parsing strings into their respective data types.
Here's a summary table highlighting the pros and cons:
Method | Pros | Cons |
---|---|---|
int(string) , float(string) | Built-in, efficient, clear error handling | Limited to specific number formats |
eval() | Can handle expressions (use with caution) | Security risks, potential for arbitrary code execution |
Regular expressions | Flexible for complex number formats | Requires expertise in regular expressions |
ast.literal_eval() | Safer alternative to eval() for literals | Limited to basic data types |
Specialized libraries | High-precision decimal calculations | Might be overkill for simple parsing needs |
Remember, the built-in int()
and float()
functions are typically the most straightforward and efficient choices for basic parsing. Use alternative methods only when their specific functionalities are required.
python parsing floating-point
Beyond the Basics: Understanding Hash Tables and Python Dictionaries
Here's a breakdown of the concept with examples:Hash Tables:Imagine a library with books stored on shelves. Finding a specific book would be slow if you had to check each book on every shelf...
Simplifying Database Interactions with SQLAlchemy: Getting Started
Understanding the Problem:Goal: To retrieve a list of table names from a MySQL database using SQLAlchemy, a Python library that streamlines database interactions...
Taming the Timestamp: Solutions for Comparing Datetimes Across Time Zones (Python/Django)
Understanding the Error:In Python, Django, and other frameworks, date and time operations rely on the datetime module. This module allows representing dates and times as datetime objects...
Choosing the Right Tool for the Job: A Comparison of dot() and @ for NumPy Matrix Multiplication
Basics:numpy. dot(): This is the classic NumPy function for matrix multiplication. It can handle a variety of input types...