Runtime Magic: Dynamically Modifying Alembic Configuration for Advanced Use Cases
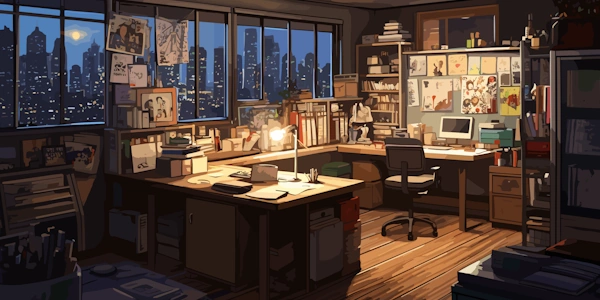
Problem:
In Alembic, a database migration tool for SQLAlchemy, the standard approach for storing database connection information is in a file named alembic.ini
. However, there are situations where you might want to store the connection string (which includes sensitive information like username and password) outside of this file. This could be for security reasons, flexibility in configuration, or integration with other systems.
Is it possible to do this?
Yes, here are a few effective methods:
Environment Variables:
- Store the connection string in an environment variable, accessible using
os.environ.get()
. - Example code in your
env.py
file:
import os
from alembic import context
config = context.config
config.set_main_option("sqlalchemy.url", os.environ.get("DATABASE_URL"))
Separate Configuration File:
- Create a separate configuration file (e.g.,
config.py
) to store sensitive information.
from config import SQLALCHEMY_DATABASE_URL
from alembic import context
config = context.config
config.set_main_option("sqlalchemy.url", SQLALCHEMY_DATABASE_URL)
Programmatic Configuration:
- Construct a
Config
object directly in Python, setting the connection string usingset_main_option()
. - Example code:
from alembic import Config
config = Config()
config.set_main_option("sqlalchemy.url", "postgresql://user:password@host:port/database")
Modifying Configuration at Runtime:
- Use
config.set_section_option()
to modify values in specific sections of thealembic.ini
file at runtime.
Key Considerations:
- Security: Choose a method that aligns with your security practices.
- Clarity: Ensure clear documentation for configuration setup.
- Testing: Test your chosen method thoroughly to ensure it works as expected.
Additional Solutions:
- Encrypted Secrets Manager: Store credentials in an encrypted secrets manager and retrieve them programmatically.
- Custom Configuration Files: Employ custom configuration file formats (e.g., JSON, YAML) parsed using appropriate libraries.
python sqlalchemy alembic
Keeping Your Code Clean: Strategies for Organizing Python Classes Across Files
Multiple Classes in a Single File:This is the simplest approach. You can define multiple classes within a single Python file (.py). It works well for small projects or when classes are tightly coupled and work together for a specific purpose...
Mapping Self-Referential Relationships in SQLAlchemy (Python)
I'd be glad to explain how to map a self-referential one-to-many relationship in SQLAlchemy using the declarative form for Python:...
Conquering Character Chaos: How to Handle Encoding Errors While Reading Files in Python
Understanding the Error:This error arises when you try to read a text file using the 'charmap' encoding, but the file contains characters that this encoding cannot represent...
Troubleshooting "Error during download: 'NoneType' object has no attribute 'groups'" in gdown
Error Breakdown:'NoneType' object: This indicates that a variable or expression you're trying to use doesn't hold a value...