Taming the Multidimensional Beast: A Practical Guide to NumPy Dimensions and Axes
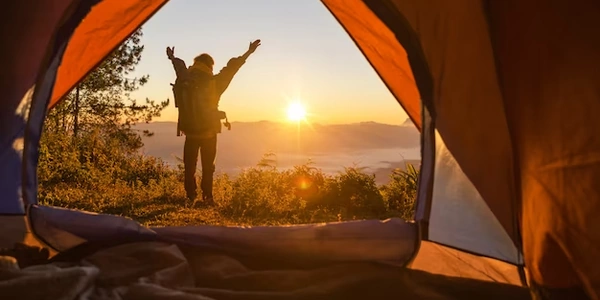
Dimensions and Axes in NumPy: Concepts and Operations
In NumPy, arrays are fundamental data structures that can hold multiple values, organized in a grid-like structure. The number of directions in which this grid extends defines the array's dimensionality, with each direction called an axis. Just like the x, y, and z axes represent positions in 3D space, axes in NumPy arrays serve as indices to access and manipulate individual elements.
Understanding Dimensions:
- 0-Dimensional (Scalar): A single value, treated as a 1D array with size 1 (e.g.,
np.array(5)
). - 1-Dimensional (Vector): A sequence of values along a single axis (e.g.,
np.array([1, 2, 3])
). - 2-Dimensional (Matrix): A table of values with rows and columns (e.g.,
np.array([[1, 2], [3, 4]])
). - 3-Dimensional (Tensor): A cube of values with layers, rows, and columns (e.g.,
np.array([[[1, 2], [3, 4]], [[5, 6], [7, 8]]])
). - Higher Dimensions: NumPy supports arrays with more than three dimensions, though they become less intuitive to visualize for humans.
Axis Indexing and Operations:
- Zero-based: Axes are always numbered from 0, making the first axis the outermost direction.
- Accessing Elements: Use square brackets and axis indices (within parentheses) to select specific elements (e.g.,
arr[0]
,arr[1, 2]
). - Reshaping: Change the layout of an array by specifying new dimensions using
arr.reshape((new_shape))
. - Broadcasting: Perform element-wise operations on arrays with compatible shapes, automatically broadcasting smaller arrays to match larger ones.
- Axis-Specific Operations: Certain functions accept an
axis
argument to specify which axis to operate along (e.g.,np.sum(arr, axis=0)
,np.mean(arr, axis=1)
).
Key Points and Common Issues:
- Clarity: Be mindful of your audience's level of expertise when explaining concepts.
- Conciseness: Strike a balance between comprehensiveness and brevity.
- Structure: Organize your explanation logically, providing step-by-step examples.
- Visuals: Consider using diagrams or animations to make concepts more intuitive, especially for higher dimensions.
- Error Handling: Address common mistakes (e.g., out-of-bounds indexing) and how to avoid them.
- Real-World Applications: Provide real-world examples of how dimensions and axes are used in data analysis, image processing, scientific computing, etc.
By combining these elements, you can create a clear, engaging, and informative explanation that addresses the core issues and helps beginners effectively grasp these fundamental concepts in NumPy.
python numpy
Navigate with Ease: A Beginner's Guide to Directory Manipulation in Python (with Django Examples)
Understanding the Problem:Python's os. path module: Provides functions for working with file and directory paths in a platform-independent way...
Three's a Crowd? Not in pandas: Efficiently Combine DataFrames for Powerful Analysis
Three-Way Joins in pandas: Combining Data from Multiple DataFramesIn data analysis, often you'll need to combine information from multiple sources represented as separate DataFrames in pandas...
Best Practices for Fetching Single Database Results in Python: A Detailed Comparison of .one() and .first()
Purpose:Both . one() and . first() are used to fetch a single row from a database query.However, they differ in how they handle cases with multiple or no results...
Bridging the Gap: Integrating Matplotlib with TensorBoard for Enhanced Data Exploration
Understanding the Approach:TensorBoard's Image Dashboard: This built-in feature is designed to visualize image data. While it primarily handles tensors representing images...